list
1. List Structure
- Use square brackets to represent lists.
- Elements inside the list are separated by commas.
- Note that it is in the English input method.
student1 = ['lilei', 18, 'class01', 201901]
student2 = ['hanmeimei', 19, 'class02', 201902]
The mutability of a list: you can modify the content inside the list.
- Convert a string to a list
string_to_list = list("Bornforthis")
print(string_to_list)
# output
['B', 'o', 'r', 'n', 'f', 'o', 'r', 't', 'h', 'i', 's']
2. Accessing Elements in a List
2.1 Composition of List Indices
In programming languages, the first position is usually numbered 0.
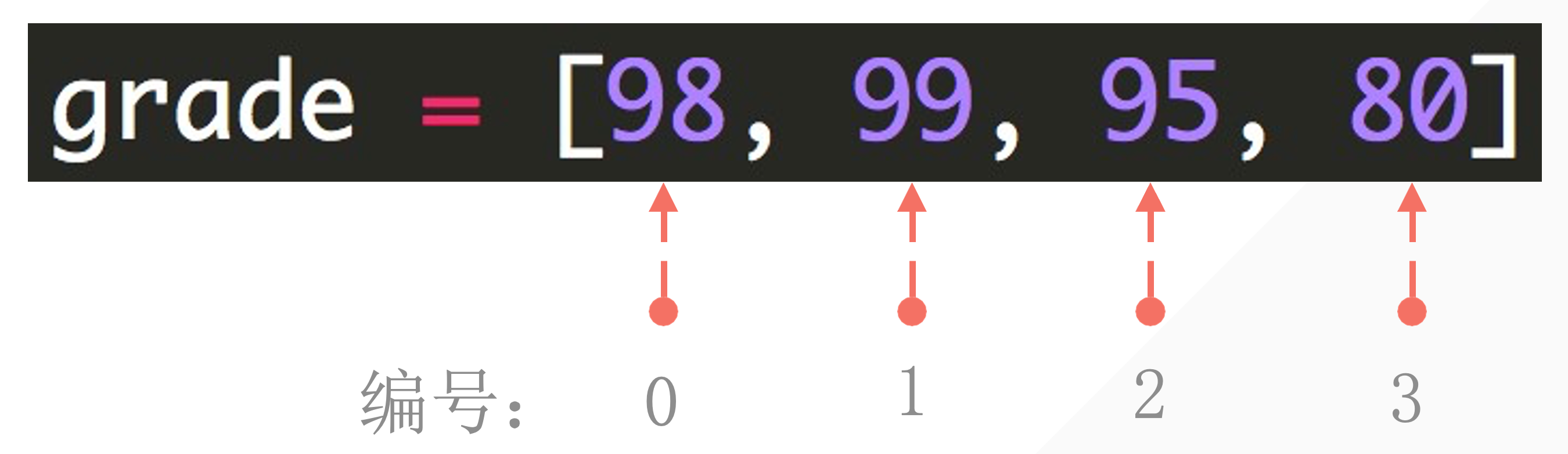
2.2 Extracting a Single Element
The number inside the square brackets specifies the element's position.
grade = [98, 99, 95, 80]
print(grade[0]) # 98
print(grade[0] + grade[3]) # 178
2.3 Getting a Range of Elements in a List
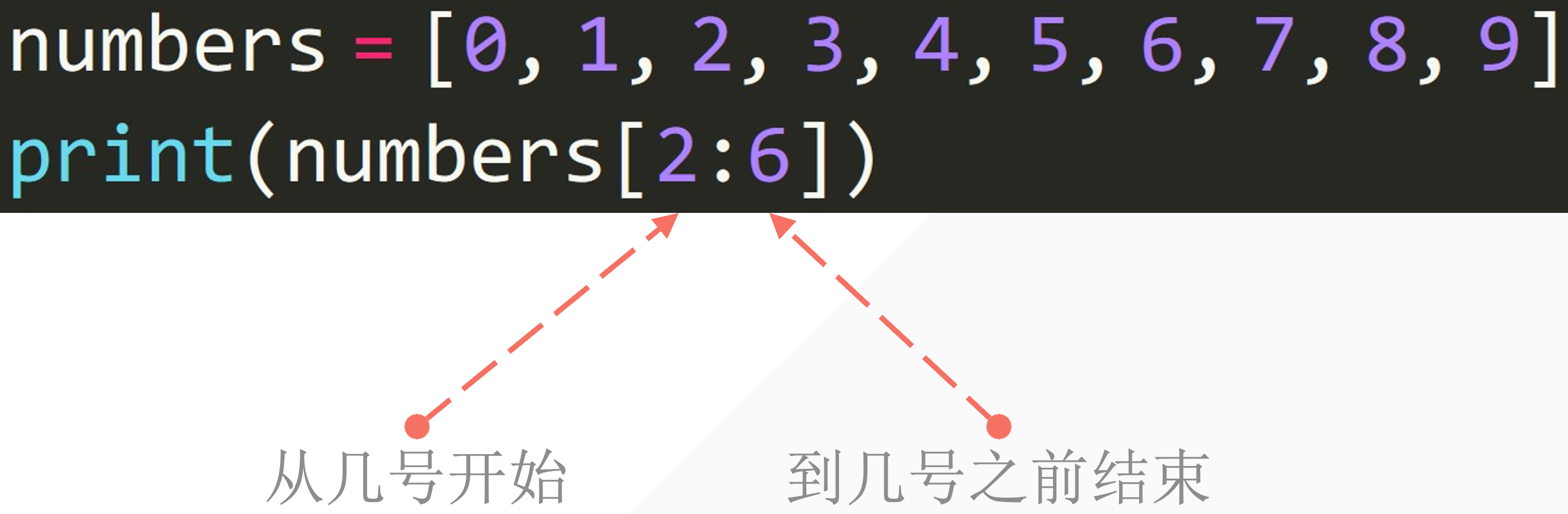
- Inside the square brackets, use start_position:end_position.
- Note: It does not include the element at the end position.
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(numbers[2:6]) # [2, 3, 4, 5]
2.4 Getting Elements with a Specific Interval in a List
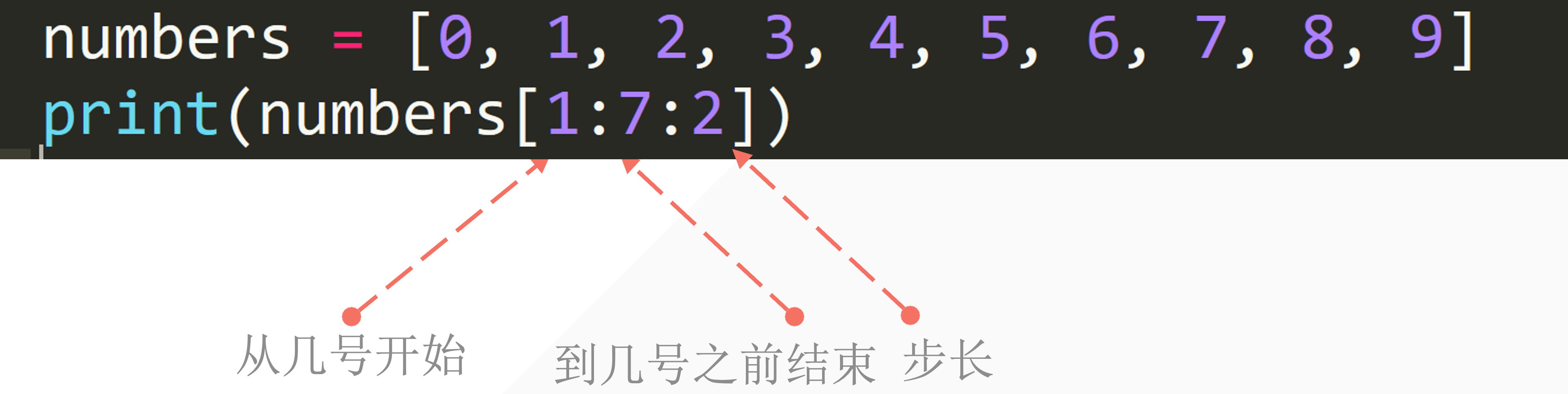
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(numbers[1:7:2]) # [1, 3, 5]
grade = [98, 99, 95, 80]
print(grade[1])
print(grade[0], grade[2])
print(grade[0:4]) # The third position defaults to +1, so it must be changed to -1 when reversed
print(grade[-1:-4:-1]) # Reverse the list
3. List Slicing Assignment
:::tab
@tab Code
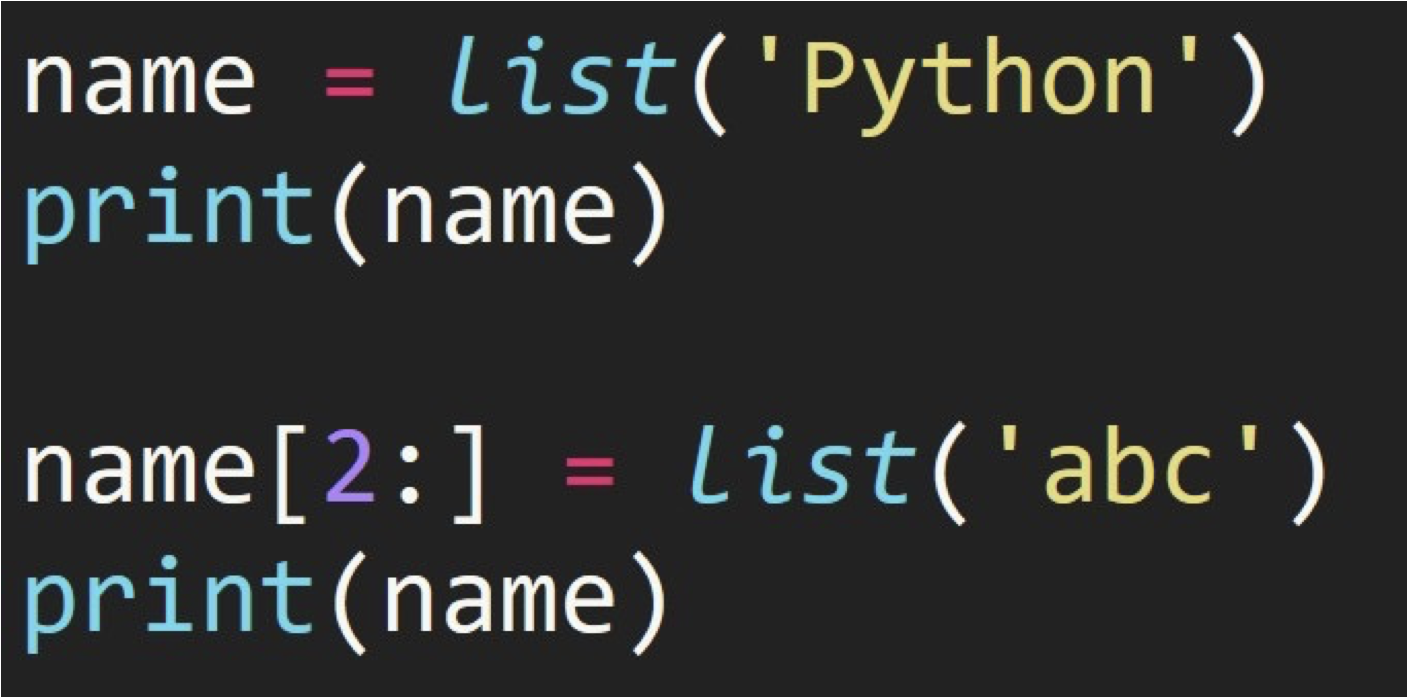
@tab Think
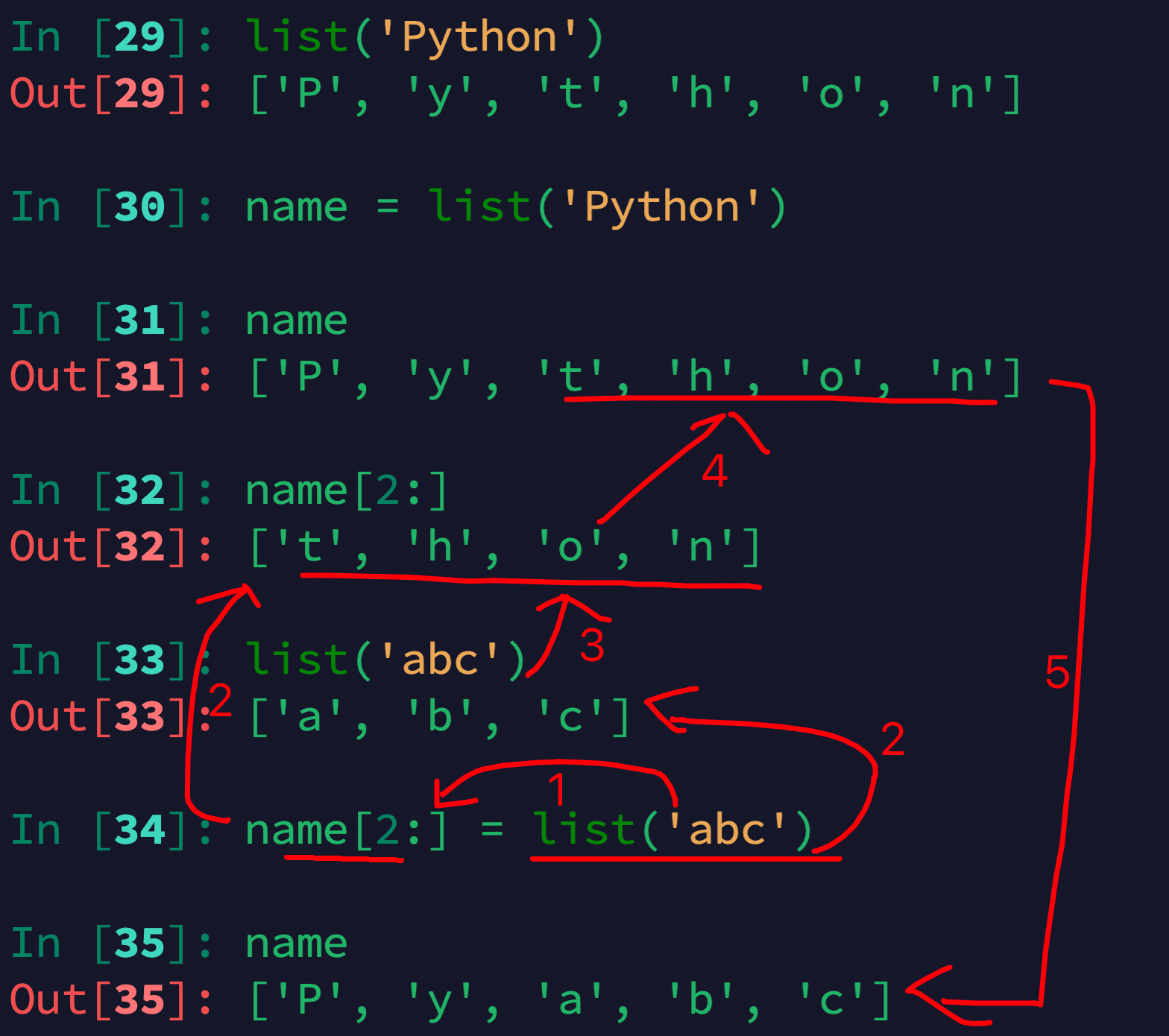
:::
In [1]: name = list('Python')
In [2]: name
Out[2]: ['P', 'y', 't', 'h', 'o', 'n']
In [3]: name[2:]
Out[3]: ['t', 'h', 'o', 'n']
In [4]: list('abc')
Out[4]: ['a', 'b', 'c']
In [5]: name[2:]=list('abc')
In [6]: name
Out[6]: ['P', 'y', 'a', 'b', 'c']
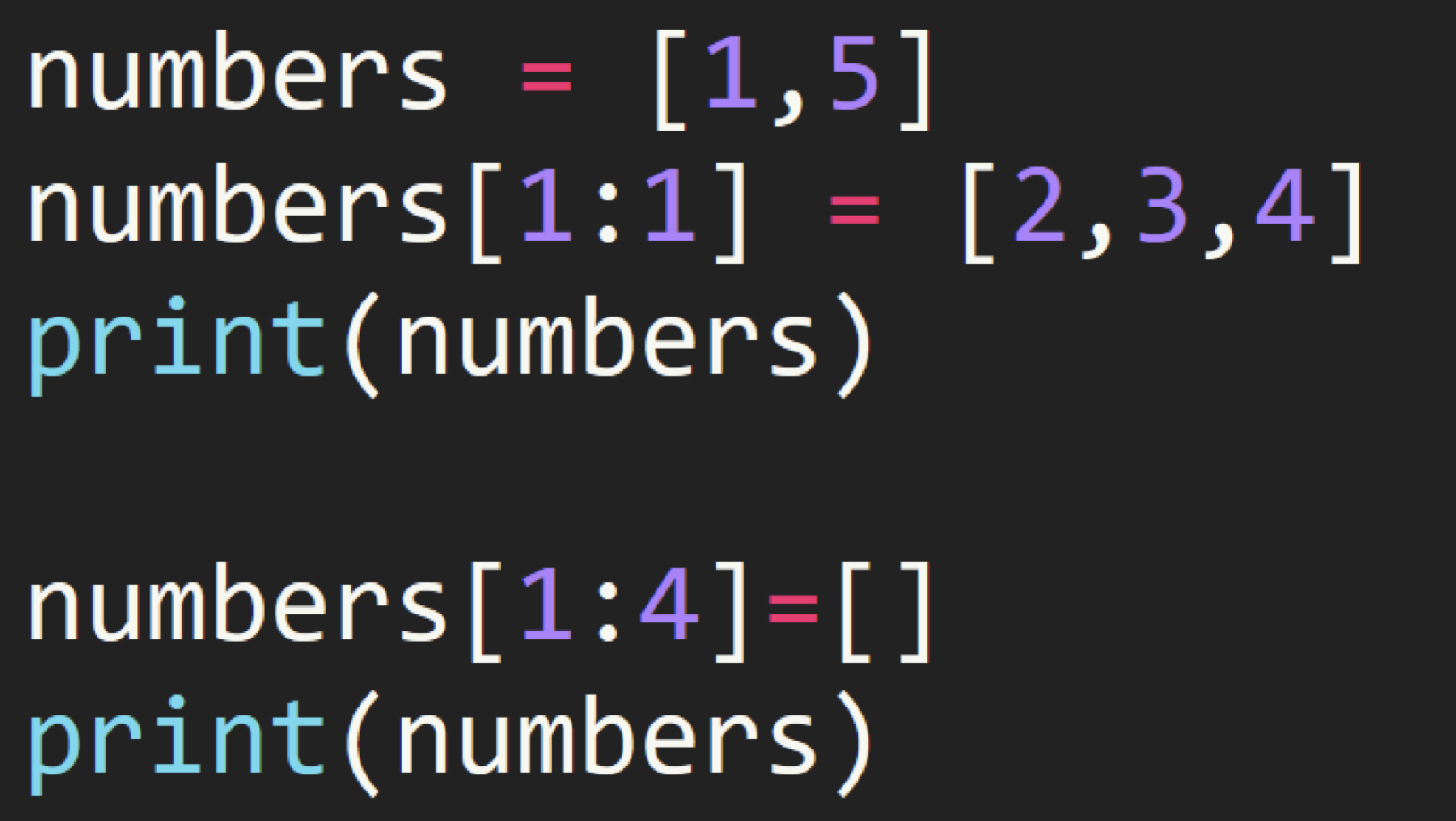
In [7]: numbers = [1, 5]
In [8]: numbers[1:1]
Out[8]: [] # Cannot access 5
In [9]: numbers[1:1] = [2, 3, 4]
In [10]: numbers
Out[10]: [1, 2, 3, 4, 5]
In [11]: numbers[1:4] = []
In [12]: numbers
Out[12]: [1, 5]
4. Trying It Out
Get user input for two values: one is the position to insert, and the other is the value to be inserted at that position.
Given the following list:
numbers = [1, 2, 3, 5, 6]
Example:
Enter position: 3
Enter value: 4
[1, 2, 3, 4, 5, 6]
numbers = [1, 2, 3, 5, 6]
end = len(numbers)
position = int(input(f"Enter position (enter a value from 0 to {end}):>>> "))
value = int(input("Enter value:>>> "))
numbers[position: position] = [value]
print(numbers)
numbers = [1, 2, 3, 5, 6]
end = len(numbers)
position = int(input(f"Enter position (enter a value from 0 to {end}):>>> "))
value = int(input("Enter value:>>> "))
result = numbers[:position] + [value] + numbers[position:]
print(result)
.insert(index, element)
5. Inserting Elements at a Specific Position - .insert(index, element)
is a basic method for lists, used to insert an element at a specified position.
Its basic syntax is:
list.insert(index, element)
index
: Specifies the position to insert the element. The index starts from 0. If the specified index is beyond the current length of the list (no error will be raised), the element will be added to the end of the list.element
: This is the element you want to insert into the list.
numbers = [1, 2, 3, 5, 6]
numbers.insert(3, 4)
print(numbers)
# output
[1, 2, 3, 4, 5, 6]
6. List Length
To get the length of a list, use len()
:
numbers = [1, 2, 3, 5, 6]
print(len(numbers))
# output
5
7. Modifying Elements in a List
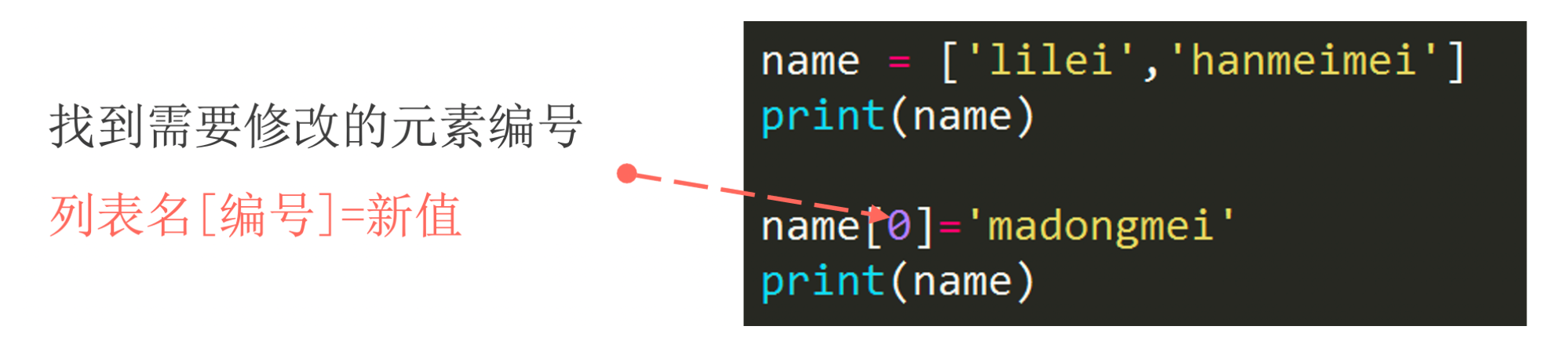
- Modifying a Single Element
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers[0] = "xxx"
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: ['xxx', 2, 3, 5, 6]
- Modifying Multiple Elements
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers[0:3] = ["xxx", "yyy", "zzz"]
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: ['xxx', 'yyy', 'zzz', 5, 6]
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers[0:5] = ["xxx", "yyy"]
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: ['xxx', 'yyy']
- Multiple modifications with a string
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers[0:3] = 'bornforthis'
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: ['b', 'o', 'r', 'n', 'f', 'o', 'r', 't', 'h', 'i', 's', 5, 6]
- Objects that can be used for multiple element modification:
- List
- Tuple
- Set
- String
- Dictionary (with keys)
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers[0:3] = {"a": 1, "b": 8}
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: ['a', 'b', 5, 6]
- Objects not allowed for multiple element modification:
- Boolean
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers[0:3] = True
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
Traceback (most recent call last):
File "/Users/gaxa/Coder/Pythonfile/data_type.py", line 3, in <module>
numbers[0:3] = True
~~~~~~~^^^^^
TypeError: can only assign an iterable
8. Adding Elements to a List
.append()
8.1 Adding a Single Element - 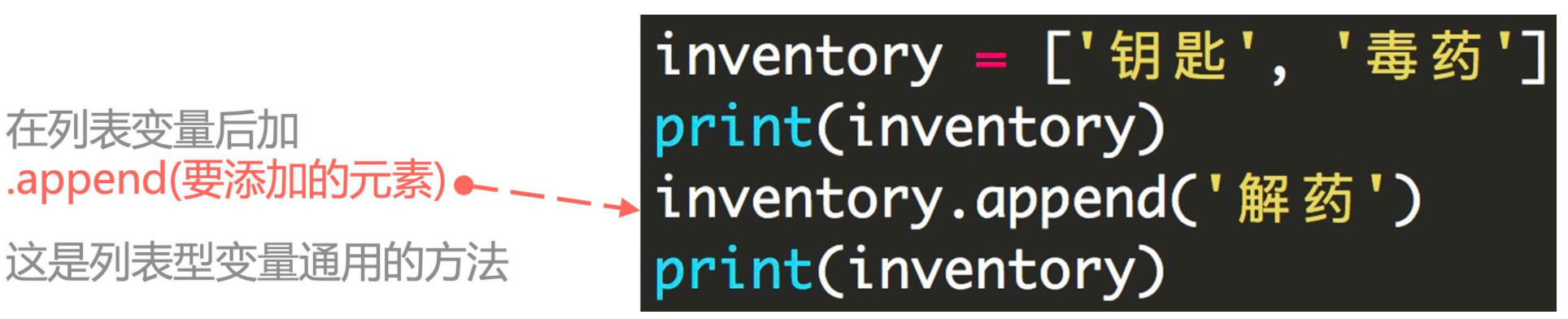
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers.append("aaa")
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: [1, 2, 3, 5, 6, 'aaa']
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers.append(["aaa", "bbb"])
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: [1, 2, 3, 5, 6, ['aaa', 'bbb']]
# It will put the entire list inside; does not support adding multiple elements
8.2 Adding Multiple Elements
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers.extend(["aaa", "bbb"])
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: [1, 2, 3, 5, 6, 'aaa', 'bbb']
9. Deleting Elements from a List
del
9.1 del
is used to specify individual or multiple elements to be deleted from the list.
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
del numbers[2]
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: [1, 2, 5, 6]
If not specifying elements to delete, it will delete the entire variable.
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
del numbers
print("after:", numbers)
# output
Traceback (most recent call last):
File "/Users/gaxa/Coder/Pythonfile/data_type.py", line 4, in <module>
print("after:", numbers)
^^^^^^^
NameError: name 'numbers' is not defined
before: [1, 2, 3, 5, 6]
pop()
9.2 pop()
function by default deletes the last element from the list, and it can also take an argument to specify the index of the element to be removed.
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers.pop()
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: [1, 2, 3, 5]
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers.pop(0)
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: [2, 3, 5, 6]
remove()
9.3 remove()
is used to specify the removal of a particular element from the list. For example, remove('aiyc')
specifies the removal of the 'aiyc'
element from the list.
If there are duplicates, only one instance will be removed.
numbers = [1, 2, 3, 5, 6]
print("before:", numbers)
numbers.remove(2) # element 2 not the 3rd element
print("after:", numbers)
# output
before: [1, 2, 3, 5, 6]
after: [1, 3, 5, 6]
10. Combining Two Lists
Simply use the plus sign.
numbers1 = [1, 2, 3, 5, 6]
numbers2 = [10, 20, 30]
print(numbers1 + numbers2)
# output
[1, 2, 3, 5, 6, 10, 20, 30]
Value in Sequence
11. Checking if an Element Exists in a List - 
numbers = [1, 2, 3, 5, 6]
print(1 in numbers)
print(10 in numbers)
# output
True
False
.count()
12. Getting the Repeat Count of an Element in a List - numbers = [1, 2, 1, 1, 3, 5, 6]
print(numbers.count(1))
# output
3
.index()
13. Get the First Occurrence Position of an Element in a List - Use list.index(element) to obtain the position. If the element is not in the list, an error will be raised.
numbers = [1, 2, 1, 1, 3, 5, 6]
print(numbers.index(1))
# Output
0
14. Sorting a List
sort(reverse=False)
14.1 list.sort()
arranges elements in the list in ascending order and modifies the list directly. If reverse=True
is specified, the list is sorted in descending order.
numbers = [1, 2, 1, 1, 3, 5, 6]
numbers.sort()
print(numbers)
numbers.sort(reverse=True)
print(numbers)
# Output
[1, 1, 1, 2, 3, 5, 6]
[6, 5, 3, 2, 1, 1, 1]
sorted(list, reverse=False)
14.2 sorted(list, reverse=False)
sorts the list in ascending order, leaving the original list unchanged and returning a new list. reverse
is set to False by default, and if set to True, it returns the list in descending order.
numbers = [1, 2, 1, 1, 3, 5, 6]
new_numbers = sorted(numbers)
print(new_numbers)
# Output
[1, 1, 1, 2, 3, 5, 6]
- 0
- 0
- 0
- 0
- 0
- 0