Numeric Type
1. Characteristics of Numeric Types
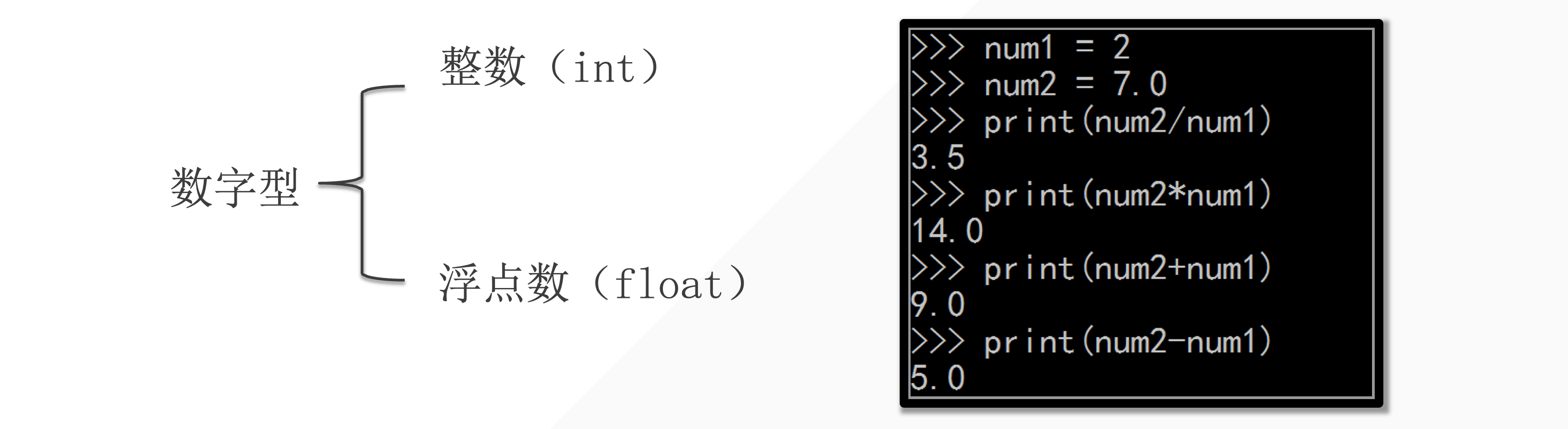
In [2]: 1+1
Out[2]: 2
In [3]: 1+1.0
Out[3]: 2.0
In [4]: 9-1
Out[4]: 8
In [5]: 9-1.0
Out[5]: 8.0
In [6]: 2*2
Out[6]: 4
In [7]: 2*2.0
Out[7]: 4.0
In [8]: 9/3
Out[8]: 3.0
In [9]: # If one of the numbers is float, the result will be float (highest priority)
In [10]: # Division involves precision issues, so the result is a float
Patterns
If one of the numbers is a float, the result will be a float (highest priority).
Division involves precision issues, so the result type is a float.
2. Arithmetic Operators
Arithmetic Operators: Used for arithmetic calculations.
Operator | Description | Example |
---|---|---|
+ | Addition operator | 1 + 1 = 2 |
- | Subtraction operator | 2 - 1 = 1 |
* | Multiplication operator | 2 * 3 = 6 |
/ | Division operator | 9 / 3 = 3.0 |
** | Exponentiation operator | 2 ** 3 = 8 |
% | Modulus operator, calculates remainder | 9 % 2 = 1 |
// | Floor division operator, calculates quotient and removes decimal part | 9 // 2 = 4 |
Note: 9 / 2 = 4......1
3. A Little Practice: Numeric Transformation
Suppose you have a two-digit integer, and you need to generate two new numbers based on the following rules:
- The first new number is the sum of the individual digits of the original number.
- The second new number is the reversal of the original number (e.g., if the original number is 21, the reversed number is 12).
Write Python code to implement the above requirements.
a = 12
a0 = a // 10
a1 = a % 10
b = a0 + a1
c = 10 * a1 + a0
print(b, c)
# output
3 21
Input:
An integer num
(10 ≤ num ≤ 99)
Output:
Two integers or an error message string.
Example:
Suppose the input number num
is 91, then your code should output two numbers: 10 (sum of 9 and 1) and 19 (reversed form of 91).
Suppose the input number num
is 26, then your code should output two numbers: 8 (sum of 2 and 6) and 62 (reversed form of 26).
Suppose the input number num
is 18, then your code should output two numbers: 9 (sum of 1 and 8) and 81 (reversed form of 18).
4. Comparison Operators: Comparing the Values
Operator | Description | Example |
---|---|---|
> | Checks if the first operand is greater than the second | print(1 > 2) |
< | Checks if the first operand is less than the second | print(1 < 2) |
>= | Checks if the first operand is greater than or equal to the second | print(3 >= 3) |
<= | Checks if the first operand is less than or equal to the second | print(3 <= 4) |
== | Checks if the two operands are equal | print(2 == 2) |
!= | Checks if the two operands are not equal | print(2 != 1) |
Try the examples above and see the results?
print(1 > 2)
print(1 < 2)
print(3 >= 3)
print(3 <= 4)
print(2 == 2)
print(2 != 1)
# output
False
True
True
True
True
True
5. Assignment Operators
Operator | Description | Example |
---|---|---|
= | Assigns the value on the right to the variable on the left | a = 1 |
+= | a += b is equivalent to a = a + b | a += 10 |
-= | a -= b is equivalent to `a |
= a - b |
a -= 10 | |
*= |
a *= bis equivalent to
a = a * b |
a *= 10 | |
/= |
a /= bis equivalent to
a = a / b |
a /= 10 | |
**= |
a **= bis equivalent to
a = a ** b |
a **= 10 | |
//= |
a //= bis equivalent to
a = a // b |
a //= 10` |
a = 1
a += 10
a -= 10
a *= 10
a /= 10
a **= 10
a //= 10
print(a)
# output
0.0 # Division results in a float
# Assignment operator form
a = 1
a += 10
print(a) # Outputs 11
# Regular form
a = 1
a = a + 10
print(a) # Outputs 11
a = 4 // 2 # Integer result
b = 4.5 // 2 # Float result
print(a, b)
# output
2, 2.0
7.3.1 Sum and Difference of Numbers:
Write a Python code snippet that creates two numbers a
and b
, calculates and prints their sum and the result of subtracting a
from b
.
Code Template
a = 10
b = 5
Sum = a + b
Difference = a - b
print(Sum, Difference)
# Test
assert Sum == a + b
assert Difference == a - b
Output Example:
Sum: 8
Difference: 2
7.3.2 Multiplication and Division of Numbers
Write a Python code snippet that creates two numbers x
and y
, calculates and prints their product and division result.
Code Template
x = 10
y = 5
product = x * y
division = x / y
print(product, division)
# Test
assert product == x * y
assert division == x / y
Output Example:
Product: 12
Division: 3.0
7.3.3 Remainder and Power Operation
Write a Python code snippet that creates two numbers m
and n
, calculates and prints the remainder of m
divided by n
and m
raised to the power of n
.
Code Template
m = 10
n = 5
floor_div = m // n
power = m ** n
print(floor_div, power)
# Test
assert remainder == m % n
assert power == m ** n
**Output Example:
Sure, here's the translation of the provided text into English in Markdown format:
**Code Exercise: Comparisons**
```python
Remainder: 1
Power: 81
7.3.4 Comparison Operations
Write a Python code snippet that takes two numbers p
and q
as input, compares their sizes, and prints the corresponding result (greater than, less than, equal to).
Code Template
p = 10
q = 5
if p > q:
print(p, " is greater than ", q)
elif p < q:
print(p, " is less than ", q)
else:
print(p, " is equal to ", q)
if ______:
print(f"{p} is greater than {q}")
assert p > q
elif ______:
print(f"{p} is less than {q}")
assert p < q
else:
print(f"{p} is equal to {q}")
assert p == q
Output Example:
4 is less than 5
- 0
- 0
- 0
- 0
- 0
- 0